Rhizome's Programs
The name is generic, but Programs are what Rhizome uses to modularize separate bits of functionality.
Rails developers often want ways to cleanly modularize code, but the most common options like creating code in separate gems or Rails engines is too clunky. It's almost never something you see done in practice because it imposes too much overhead or muddies the boundaries between the main framework and your business logic.
Program Structure
Assume the following examples are located under
app/programs/customers
. app/programs
is the expected place to make sure that everything gets auto-loaded by Rails without having to bend over backwards or break Rails' expectations.
The Definition
Every Rhizome program is required to have a definition file in your program
called
definition.rb
which is just Ruby code. Your definition will be run automatically
whenever you save it in order to create the Steps that make up your program. You can think of Steps as forms with Fields (but they can also be dumb data objects). For example, a simple program might look like this:
module Customers
class Definition
def self.load
prog = Program.new(name: "Customers", account: $default_account)
# create or update a Step with a key and kind fields "Customer"
prog.def_blueprint("Customer", {
fields: [
{name: "First Name", kind: "text", searchable: true},
{name: "Last Name", kind: "text", searchable: true}
# ... more fields here
]
})
end
end
end
This already provides you with loads of good stuff like a customer form, JSON API, search, a CSV uploader, audited history, and more. Here we just show text
fields but there are many kinds of default Fields included, and you always have the ability to define more.
Commands
This directory contains the "verbs" for your program, and can contain files that are referenced from your program's definition file. For example to expand on the last example:
module Customers
class Definition
def self.load
prog = Program.new(name: "Customers", account: $default_account)
prog.def_blueprint("Customer", {
fields: [
{name: "First Name", kind: "text", required: true},
{name: "Last Name", kind: "text", required: true},
{
name: "Send to CRM",
kind: "command",
command: "customers/send_to_crm"
}
]
})
end
end
end
Which by default would give us a form like this:
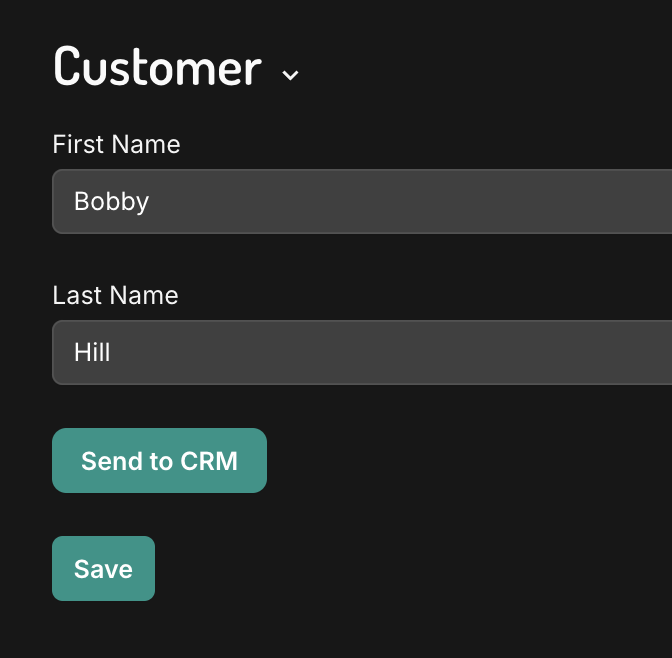
Of course, clicking "Send to CRM" without a command file defined will result in an error, so check out the docs for Commands to see how to define a command file.
Assets
Anything you'd put in your app/assets
directory in a traditional Rails app can go here. The most common uses are for images, svgs, pdfs, and vanilla javascript files. Access it using the asset_path
method or similar from ActionView::Helpers::AssetUrlHelper
.
Controllers and Routes
If you end up needing them, custom controllers can live under a controllers
directory in your program and be routed to by a routes.rb
file in the project root. For example, this documentation page itself comes from the docs
program in Rootstock, if you want to see how it's wired up. The only thing of note is that we follow Rails naming conventions so the controller should be called YourModuleName::Controllers::ControllerName
.
Views
Just like in any Rails app, you can have a separate directory in your program for views. This is handy for fields where with kind "content" or for custom routed actions (like these docs). You can also reference layout files if you put them under views/layouts
.
Note that views aren't namespaced like the rest of your code, so names will be resolved in the order they were loaded. To avoid conflicts, a best practice is to always reference the preceding directory name under views, for example: render "customers/show"
. You can also name the top directory, e.g. "customers", to something more unique if there's a conflict.
Tests
Rhizome will automatically run files within your program that end in *test.rb
. You can run them with ./bin/rails test:programs
or just by running a test/unit
test file directly.
Anything Else
Whatever you want to reference manually like schema files, background jobs, test fixtures, sample uploads, etc. can be placed in your programs.
We find this to be a much better way of modularizing our Rails apps, and in the case of Rhizome Compliance, it allows us to sell access to customized or proprietary programs a la carte.